Creating an MP3 Playlist in HTML: A Step-by-Step Guide for Beginners
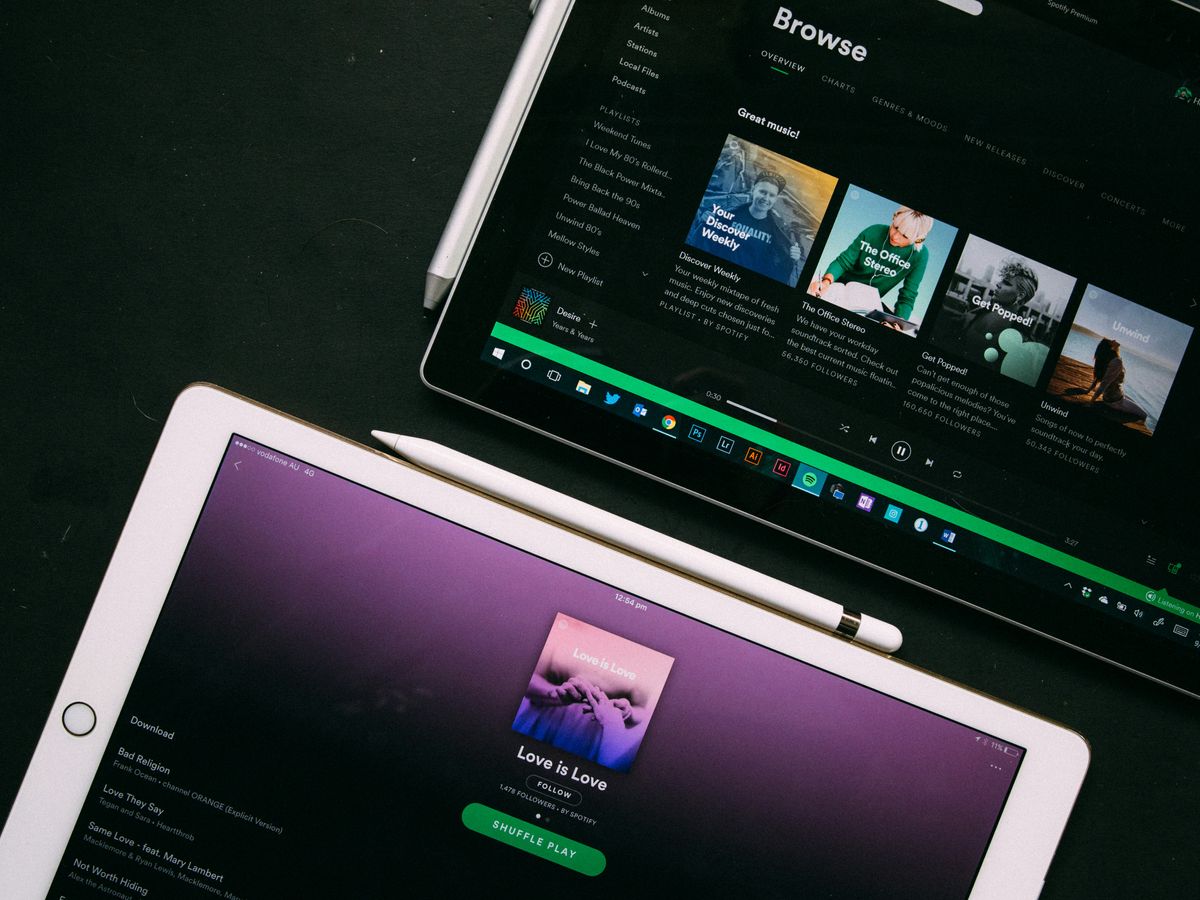
Creating an MP3 playlist in HTML can seem daunting, especially if you're new to web development. But don't worry! This guide will walk you through the process step by step, making it easy to add your favorite audio tracks to your website. By the end, you'll have a functional and stylish MP3 playlist that you can share with your visitors.
Key Takeaways
- Start by understanding the HTML audio element and its attributes.
- Customize your audio player using CSS to match your site's style.
- Add tracks by uploading files or linking to online sources.
- Enhance functionality with features like autoplay, shuffle, and repeat.
- Test your playlist across different browsers to ensure compatibility.
Creating Your First MP3 Playlist
Let's get started building our very own MP3 playlist! It might seem a little intimidating at first, but trust us, it's easier than you think. We'll break it down into simple steps so we can all follow along. The goal is to have a basic, functional playlist that we can then customize and enhance later on. Think of it as building blocks – we need a solid foundation before we can add all the cool features.
Understanding the Basics of HTML Audio
Okay, so before we jump into coding, let's quickly cover the basics of HTML audio. The main thing we need to know is the <audio>
tag. This tag is what allows us to embed audio files directly into our web pages. It's like the <img>
tag, but for sound! We can specify the audio source, add controls for playback, and even set things like autoplay and looping. The HTML audio element is a powerful tool.
Setting Up Your HTML Document
First things first, we need an HTML document to work with. If you already have one, great! If not, no worries, just create a new file and save it as something like playlist.html
. Here's some basic HTML to get us started:
<!DOCTYPE html>
<html>
<head>
<title>My MP3 Playlist</title>
</head>
<body>
<!-- Our playlist will go here -->
</body>
</html>
This is the bare minimum – a basic HTML structure with a title. We'll be adding our audio player inside the <body>
tags.
Adding the Audio Element
Now for the fun part! Let's add the <audio>
element to our HTML document. Inside the <body>
tags, we'll add the following code:
<audio controls>
<source src="song.mp3" type="audio/mpeg">
Your browser does not support the audio element.
</audio>
Let's break this down:
controls
: This attribute adds the default browser controls to our audio player (play, pause, volume, etc.).<source src="song.mp3" type="audio/mpeg">
: This specifies the source of our audio file. Make sure to replacesong.mp3
with the actual name of your MP3 file. Thetype
attribute tells the browser what type of file it is.Your browser does not support the audio element.
: This is a fallback message that will be displayed if the user's browser doesn't support the<audio>
tag. It's always good to have a backup!
At this point, if you open your playlist.html file in a browser, you should see a basic audio player with controls. If you don't hear anything, double-check that your song.mp3 file is in the same directory as your HTML file, and that the file name is correct. Also, make sure your browser supports the MP3 format.
And that's it! We've created our first MP3 playlist. Okay, it's not much of a playlist yet, but it's a start. Next, we'll look at how to add more tracks and customize the player to make it look and function the way we want.
Customizing Your MP3 Playlist
Okay, so you've got a basic MP3 playlist up and running. Now, let's make it look and feel awesome! Customization is where you can really inject your personality and make the player fit seamlessly into your website's design. It's all about creating a user experience that's both functional and visually appealing. Let's get started!
Choosing the Right Player Style
First things first, think about the overall vibe you're going for. Do you want something sleek and modern, or maybe something more retro? There are tons of ways to style your player, and the right choice can make a big difference. Consider the color scheme, font, and overall layout.
- Minimalist: Clean lines, simple controls, and a focus on usability.
- Modern: Bold colors, smooth animations, and a responsive design.
- Retro: Vintage fonts, classic button styles, and a nostalgic feel.
Think about where the audio player will live on your site. Is it for a personal blog, a professional portfolio, or something else entirely? The context matters!
Styling with CSS for a Unique Look
CSS is your best friend when it comes to customization. You can tweak just about anything, from the colors of the buttons to the font used for the track titles. Don't be afraid to experiment and try out different things. Here's a simple example of how you might change the color of the play button:
.play-button {
background-color: #4CAF50; /* Green */
border: none;
color: white;
padding: 10px 20px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 16px;
margin: 4px 2px;
cursor: pointer;
}
Remember to link your CSS file to your HTML document using the <link> tag in the <head> section. This is how your styles will actually be applied to the player.
Adding Cover Images and Backgrounds
Adding cover images to your tracks can really make your playlist pop. It gives users a visual cue and makes the whole experience more engaging. You can also add a background to the player itself to tie it in with the rest of your site. Here's how you might structure your HTML to include cover images:
- Make sure each track has a corresponding image file (e.g.,
track1.jpg
,track2.jpg
). - Update your HTML to include an
<img>
tag for each track, linking to the correct image file. - Use CSS to style the images and position them nicely within the player.
Here's a basic example:
<div class="track">
<img src="track1.jpg" alt="Track 1 Cover">
<p>Track 1 Title</p>
</div>
Backgrounds can be added using CSS as well. You can use a solid color, a gradient, or even an image. Just make sure it doesn't clash with the rest of the player's elements!
Adding Tracks to Your Playlist
Alright, so you've got the basic structure of your playlist set up. Now comes the fun part: actually adding some music! There are a few different ways to get your tracks into the playlist, and we'll walk you through the most common ones.
Uploading Audio Files
First things first, you need to have your audio files ready. Make sure they're in a format that's widely supported by browsers, like MP3. The easiest way to get your music into your playlist is to upload the audio files directly to your website's server.
Here's a simple way to organize your files:
- Create a folder specifically for your audio files (e.g., "audio" or "mp3").
- Upload all your MP3 files into this folder.
- Reference these files in your HTML code.
Linking to External Sources
Sometimes, you might not want to host the audio files yourself. Maybe you're using a service like SoundCloud or have permission to use audio from another website. In that case, you can link to external sources. Just make sure you have the right to use the audio!
When linking to external sources, you'll use the full URL of the audio file in the src
attribute of your <source>
tag. For example:
<audio controls>
<source src="https://example.com/audio/mysong.mp3" type="audio/mpeg">
Your browser does not support the audio element.
</audio>
Linking to external sources can be convenient, but keep in mind that you're relying on that external source to stay online. If the source goes down, your playlist will break. It's often more reliable to host the files yourself.
Editing Track Details
Okay, so you've got your tracks in your playlist, but what about the track names? Or maybe you want to add some extra information, like the artist or album? You can do this using JavaScript to dynamically update the playlist information. This is where things get a little more advanced, but it's worth it for a polished user experience. You can use JavaScript to manage the audio element and a list of audio files.
Here's a basic example of how you might do it:
<ul id="playlist">
<li data-src="audio/track1.mp3">Track 1 - Artist</li>
<li data-src="audio/track2.mp3">Track 2 - Artist</li>
<li data-src="audio/track3.mp3">Track 3 - Artist</li>
</ul>
And then, using JavaScript, you can grab the data-src
attribute and the text content of each li
element to populate your audio player. This gives you a lot of flexibility in how you display your track information.
Enhancing Your Playlist Functionality
Okay, so you've got a basic playlist up and running. Now, let's make it really cool. We're talking about features that will keep your listeners engaged and coming back for more. Think about things like autoplay, shuffle, and a smooth, easy-to-use interface. These are the things that separate a good playlist from a great playlist. Let's get into it!
Implementing Autoplay and Loop Features
Let's start with autoplay and loop. Autoplay can be a bit controversial – some people love it, some hate it. But when used right, it can create a great first impression. Looping is almost always a good idea, especially for background music or ambient playlists. Here's how we can think about it:
- Autoplay: Consider using it sparingly. Maybe only on certain pages or for specific playlists.
- Loop: Definitely a must-have for continuous playback.
- User Control: Always give users the option to turn autoplay and loop on or off. It's about giving them control over their experience.
Autoplay can be implemented with the autoplay attribute in the <audio> tag. Looping is just as easy with the loop attribute. But remember, user experience is key. Don't force anything on your visitors.
Adding Shuffle and Repeat Options
Shuffle and repeat are playlist staples. They add variety and keep things interesting. Shuffle randomizes the order of the tracks, while repeat lets users listen to their favorites over and over. Implementing these features usually involves a bit of JavaScript, but it's totally worth it. Think about it like this:
- Shuffle: Great for discovering new tracks in a playlist.
- Repeat One: Perfect for those songs you just can't get enough of.
- Repeat All: Keeps the playlist going without manually restarting it.
We can use JavaScript to manipulate the playlist order and control the playback behavior. It might seem complicated, but there are tons of tutorials and libraries out there to help us out. For example, we can use JavaScript to create a dynamic playlist that changes based on user preferences.
Creating a User-Friendly Interface
Finally, let's talk about the interface. A clunky, confusing interface can ruin even the best playlist. We want something that's intuitive, easy to navigate, and visually appealing. Here are a few things to keep in mind:
- Clear Controls: Make sure the play, pause, skip, and volume controls are easy to find and use.
- Playlist Display: Show the current track and the upcoming tracks in a clear, organized way.
- Mobile-Friendly: Ensure the interface looks and works great on all devices.
Feature | Description |
---|---|
Play/Pause | Starts and stops the audio playback. |
Skip | Moves to the next or previous track. |
Volume Control | Adjusts the audio volume. |
Playlist Display | Shows the list of tracks and the current track being played. |
Shuffle/Repeat | Options to randomize or repeat the playlist. |
By focusing on these elements, we can create a playlist that's not only functional but also a joy to use. And that's what it's all about, right?
Embedding Your Playlist on a Website
Okay, so you've got your MP3 playlist all set up. Now, let's get it out there for the world to hear! Embedding it on your website is easier than you might think. We'll walk you through the steps.
Copying the HTML Code
First things first, you need to grab the HTML code for your playlist. If you've been following along, you should have this ready to go. This code is what tells the browser how to display your playlist. It's like the blueprint for your music player. Make sure you copy the entire code snippet. Missing even a single character can cause problems.
Inserting Code into Your Web Page
Now, where do you put this code? Well, it depends on your website. If you're using a simple HTML editor, just open the HTML file of the page where you want the playlist to appear. Paste the code into the <body>
section, wherever you want the player to show up. If you're using a CMS like WordPress, look for the option to edit the HTML of a page or post. You might need to use a text editor widget or block to insert the code properly. For example, you can use a music plugin to easily add the audio player.
Testing Your Playlist
Alright, you've pasted the code. Now for the moment of truth: testing! Save your changes and open the web page in your browser. Does the playlist show up? Can you play the tracks? If not, double-check that you copied the HTML code correctly and that all your audio files are linked properly. Sometimes, a simple refresh of the page can do the trick. If you're still having trouble, take a look at the "Troubleshooting Common Issues" section for some tips.
Troubleshooting Common Issues
Okay, so you've built your MP3 playlist, but things aren't quite working as expected? Don't worry, we've all been there. Let's walk through some common problems and how to fix them. It's usually something simple, so don't panic!
Audio Not Playing?
This is probably the most frustrating issue. Here's a checklist to run through:
- Check your file paths: Are the paths to your MP3 files correct? Even a small typo can cause the audio not to load. Double-check the spelling and make sure the files are actually located where you think they are.
- File format: Is the file actually an MP3? Sometimes files get mislabeled. Try opening the file in a dedicated audio player to confirm it plays.
- Browser support: Some older browsers might not support certain audio codecs. Try using a more common format or updating your browser.
- Permissions: If you're running the playlist from a local file, your browser might be blocking access to the audio files for security reasons. Try running it from a simple web server (even just opening it in VS Code with Live Server can work).
Sometimes, the issue isn't with your code at all, but with the browser's security settings. Browsers are getting stricter about what they allow, especially when it comes to local files. So, before you tear your hair out, try a different browser or check your browser's console for error messages.
Playlist Not Displaying Correctly
So, the audio is playing, but the playlist looks all messed up? Let's troubleshoot:
- CSS issues: Double-check your CSS. Are there any conflicting styles that might be messing with the layout? Use your browser's developer tools to inspect the elements and see what styles are being applied.
- HTML structure: Is your HTML properly structured? Make sure all your tags are closed and nested correctly. A single unclosed
div
can throw off the entire layout. - JavaScript errors: If you're using JavaScript to dynamically generate the playlist, check the console for any errors. A JavaScript error can prevent the playlist from rendering correctly.
Browser Compatibility Problems
What works perfectly in Chrome might be a disaster in Firefox or Safari. Here's how to handle it:
- Test in multiple browsers: Always test your playlist in different browsers to identify any compatibility issues. This is the most important step.
- Use browser-specific CSS: You can use CSS hacks or browser-specific prefixes to apply different styles for different browsers. However, this can get messy, so use it sparingly.
- Consider a JavaScript library: Libraries like Plyr can help abstract away the differences between browsers and provide a consistent player experience. If you are having issues with HTML autoplay, a library might help.
Browser | Supported Audio Formats | Known Issues |
---|---|---|
Chrome | MP3, WAV, Ogg | Autoplay policies can be strict |
Firefox | MP3, WAV, Ogg | Occasional issues with certain Ogg codecs |
Safari | MP3, WAV, AAC | Can be picky about AAC encoding |
Edge | MP3, WAV, AAC | Generally good compatibility, based on Chrome |
Remember, debugging is a process of elimination. Take it one step at a time, and you'll get there!
Exploring Advanced Features
Okay, so you've got a basic playlist up and running. Now, let's crank things up a notch! We're going to look at some advanced features that can really make your playlist stand out. Think beyond just playing songs – we're talking about interactivity, dynamic content, and even tapping into external services. It might sound intimidating, but trust us, it's totally doable, and the results are worth it.
Integrating JavaScript for Interactivity
JavaScript is where the magic happens. It lets us go beyond simple HTML audio controls and add all sorts of cool features. Want to create custom buttons? Need to display track information dynamically? JavaScript is your friend. With JavaScript, we can manipulate the audio element, respond to user actions, and update the page in real-time.
Here are some ideas:
- Custom Play/Pause Buttons: Replace the default browser controls with your own styled buttons.
- Volume Control: Add a slider to adjust the volume.
- Progress Bar: Show how much of the song has played and allow users to jump to different parts.
Creating Dynamic Playlists
Static playlists are fine, but what if you want your playlist to change based on user preferences or external data? That's where dynamic playlists come in. We can use JavaScript to fetch playlist data from a server or even let users create their own playlists. Think of it like a mini-Spotify, but built by you!
Here's how you might approach it:
- Store playlist data in a JSON file or database.
- Use JavaScript to fetch the data and dynamically create the audio elements.
- Allow users to add, remove, and reorder tracks.
Using APIs for Enhanced Functionality
APIs (Application Programming Interfaces) let us tap into external services and add even more features to our playlist. Want to display song lyrics? Need to fetch album art? There's an API for that! Integrating APIs can really enhance audio players and take your playlist to the next level.
Using APIs might seem complicated at first, but there are tons of libraries and tutorials out there to help you get started. Don't be afraid to experiment and see what you can create. The possibilities are endless!
In this section, we dive into some cool advanced features that can really enhance your experience. Whether you're looking to boost your skills or just explore new tools, there's something here for everyone. Don't miss out on the full details—visit our website to learn more and unlock your potential!
Wrapping It Up
So there you have it! Creating an MP3 playlist in HTML isn’t as scary as it sounds. With just a few steps, you can have your own music player up and running on your website. Whether you went with a fancy widget or decided to code it yourself, you’ve got the tools to make your site more engaging. Now, go ahead and share your favorite tunes with the world! And hey, if you run into any hiccups, don’t hesitate to reach out for help. Happy listening!
Frequently Asked Questions
How do I make music play automatically in HTML?
You can add the autoplay option to the audio tag in your HTML code. This will start playing the music as soon as the page loads.
What is the easiest way to play audio in HTML?
To play audio, use the audio tag in your HTML. Make sure to include the controls attribute to show the play, pause, and volume buttons.
Can I add more songs to my playlist?
Yes! You can upload more audio files or link to songs from other websites to create a playlist.
What should I do if my audio isn't working?
Check if the audio file is linked correctly and that your browser supports the audio format.
How can I customize the look of my audio player?
You can use CSS to change colors, fonts, and styles to make your audio player fit your website's design.
Is it possible to make my playlist shuffle?
Yes, you can use JavaScript to add a shuffle feature that randomizes the order of the songs in your playlist.